I. Surface Level: Why Build a Tic-Tac-Toe AI? 🚀
Why bother? Simple: it's a fun way to explore how machines can think ahead, just like us. 😄 Think about it—when you avoid a hot stove after burning your hand, you're learning from feedback. 🔥
Computers? They don't get that luxury. That's where AI comes in. My Tic-Tac-Toe AI is designed to analyze every possible move, pick the best one to win, and block my sneaky attempts to beat it. It's like a mini brain plotting three steps ahead! 🧠
At its core, this is about Adversarial Search: the AI maximizes its winning chances while minimizing mine. The secret sauce? The Minimax Algorithm, paired with a clever trick called Alpha-Beta Pruning to keep things fast. Stick around for the advanced section where I break these down with code and examples—it's mind-blowing stuff. 🤯
The result? An AI that's tough to beat (but not impossible—I gave it a 5% chance to slip up so you can feel like a champ sometimes). Give it a shot and see if you can outwit it!Game Link↗ 🎉
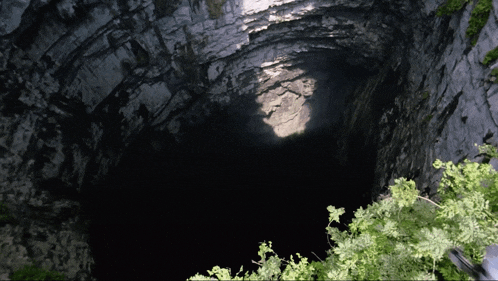
II. Advanced: The Science Behind the Smarts 💡
For my school project, I went deep into the mechanics of game AI. Here's the nitty-gritty for all you CS majors out there—complete with code, complexity analysis, and a peek at how my AI decides its next move. 📊
Purpose of Game AI 🌟
Game AI isn't just about winning—it's about simulating intelligence. In Tic-Tac-Toe, the goal is to explore every outcome and pick the optimal path, all while countering the opponent. This is where Adversarial Search shines. ✨
What's Adversarial Search? 🤔
Imagine a tug-of-war of decisions. The AI (playing ‘O') wants to win, while I (playing ‘X') try to stop it. Adversarial Search maps out all possible game states—think of it as a massive tree of “what-ifs”—and finds the best move. For Tic-Tac-Toe, that tree has up to 9! (362,880) possibilities, but we'll trim it down soon. 🌳
Game Trees & Minimax Algorithm ⚙️
A game tree is like a flowchart of every move from start to finish. Each node is a board state, and branches are moves.
The leaves? Game outcomes: win (+1 for AI), loss (-1), or draw (0). 🌿
The Minimax Algorithm navigates this tree. The AI assumes it's maximizing its score (as ‘O'), while I'm minimizing it (as ‘X'). Here's a simplified version from my code:
const minimax = (board, player, depth = 0) => {
const availSpots = board.filter((val) => val === null).length;
const winner = checkWinner(board);
if (winner === 'O') return { score: 10 - depth, index: -1 }; // AI wins
if (winner === 'X') return { score: -10 + depth, index: -1 }; // Human wins
if (availSpots === 0) return { score: 0, index: -1 }; // Draw
const moves = [];
for (let i = 0; i < board.length; i++) {
if ( !board[i]) {
board[i] = player;
const score = minimax(board, player === 'O' ? 'X' : 'O', depth + 1).score;
moves.push({ score, index: i });
board[i] = null; // Backtrack
}
}
return player === 'O'
? moves.reduce((best, move) => (move.score > best.score ? move : best))
: moves.reduce((best, move) => (move.score < best.score ? move : best));
};
- Utility Function vs. Minimax: The utility function assigns scores to end states (win/loss/draw). Minimax uses these to evaluate paths, factoring in depth to prefer quicker wins. ⏱️
- Example Tree: Picture a 3x3 board with one move made (X at center). The tree branches into 8 options for O, then 7 for X, and so on. Minimax evaluates each leaf and bubbles up the best score. 🌲
- Complexity: Without optimization, it's O(9!)—brutal for even a small game. That's where pruning saves the day. ✂️
How to Calculate Minimax Value 📊
Calculating the Minimax value involves recursively evaluating the game tree to determine the best move for the AI. Here's a step-by-step breakdown:
- Start at the current board state and generate all possible moves for the current player (e.g., 'O' for AI).
- For each move, simulate the opponent's best response (e.g., 'X' minimizing the score) by exploring the subtree.
- Assign a utility value to terminal states: +1 for an AI win, -1 for a human win, and 0 for a draw.
- Propagate the minimum or maximum score up the tree: the AI (maximizer) picks the highest score, while the human (minimizer) picks the lowest.
For example, in a board with 'X' in the center, the AI might evaluate moves leading to a win (+1) or a block (-1 if 'X' can win). The Minimax value is the maximum score from all possible moves, ensuring the AI's optimal strategy. 🧮
How the AI Decides 🎯
The AI picks the move with the highest Minimax score. In my code, `aiMove` calls `minimax` and applies the result:
const aiMove = (board) => {
let bestMove;
if (Math.random() < 0.05 && !hasMadeMistake) { // 5% mistake chance
const availSpots = board.map((val, idx) => (val === null ? idx : null)).filter(Boolean);
bestMove = availSpots[Math.floor(Math.random() * availSpots.length)];
setHasMadeMistake(true);
} else {
bestMove = minimax(board, 'O').index;
}
board[bestMove] = 'O';
setBoard([ ...board]);
};
That random 5% twist? It's my gift to you—keeps the game winnable! 🎁
Alpha-Beta Pruning: Turbocharging Minimax ⚡
Minimax checks everything, but Alpha-Beta Pruning skips branches that can't change the outcome. It tracks:
- Alpha: Best score for the maximizer (AI) so far. 📈
- Beta: Best score for the minimizer (me) so far. 📉
If a branch's score falls outside [alpha, beta], it's pruned. Here's an example:
Alpha-Beta Pruning Efficiency: This technique dramatically reduces the number of nodes evaluated. For Tic-Tac-Toe, it can cut the search space from O(9!) (362,880 possibilities) to approximately O(√9!) or around 60-70 nodes in the best case, depending on the game state, making it significantly faster! 🚀
const alphaBeta = (board, player, depth = 0, alpha = -Infinity, beta = Infinity) => {
const winner = checkWinner(board);
if (winner === 'O') return { score: 1 - depth };
if (winner === 'X') return { score: -1 + depth };
if ( !board.includes(null)) return { score: 0 };
for (let i = 0; i < board.length; i++) {
if ( !board[i]) {
board[i] = player;
const result = alphaBeta(board, player === 'O' ? 'X' : 'O', depth + 1, alpha, beta);
board[i] = null;
if (player === 'O') {
alpha = Math.max(alpha, result.score);
if (alpha >= beta) break; // Prune
} else {
beta = Math.min(beta, result.score);
if (beta <= alpha) break; // Prune
}
}
}
return { score: player === 'O' ? alpha : beta, index: -1 };
};
- Example: If the AI finds a move scoring 10, and I can force a -1 elsewhere, Alpha-Beta skips exploring worse options for me. ✅
- Efficiency: Cuts complexity to O(√9!) in the best case—way faster for Tic-Tac-Toe and critical for bigger games like Chess. 🚀
Beyond Tic-Tac-Toe 🌍
For complex games like Chess, Minimax and Alpha-Beta aren't enough alone. You'd layer in opening books, endgame databases, and heuristics (e.g., piece values) to make a beastly AI. My Tic-Tac-Toe AI is a stepping stone—simple, but packed with CS gold. 🏆
Final Thoughts 🎉
Building this AI was a blast! It's not just code—it's a window into decision-making, strategy, and optimization. Whether you're here to play or study, I hope you found something exciting. Try beating my AI (it's got that 5% flaw for you), and let me know how it goes. Happy coding! 👨💻